四角形の面積で表したものについて
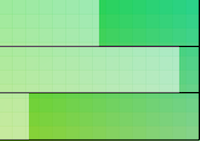
上の段から時間、分、秒を表しています。
左端は0、右端は59となるようにしました。
ソースコードはこちらから
#include "ofApp.h"
int w = 1000;
int ht = 700;
void ofApp::setup(){
ofSetFrameRate(60);
ofSetWindowShape(w, ht);
ofBackground(250, 250, 250);
ofEnableAlphaBlending();
ofEnableSmoothing();
}
void ofApp::update(){
}
void ofApp::draw(){
int s = ofGetSeconds();
int m = ofGetMinutes();
int h = ofGetHours();
ofSetColor(0, 0, 0);
ofSetLineWidth(3);
ofDrawLine(0, ht/6, w/2, ht/6);
ofDrawLine(0, ht/6 *2, w/2, ht/6 *2);
ofDrawLine(0, ht/2, w/2, ht/2);
ofDrawLine(w/2, 0, w/2, ht/2);
ofSetLineWidth(2);
ofSetColor(255, 255, 255, 100);
ofDrawRectangle(0, 0, h/24.0 * w/2, ht/6);
ofDrawRectangle(0, ht/6, m/60.0 * w/2, ht/6);
ofDrawRectangle(0, ht/3, s/60.0 * w/2, ht/6);
verdana.drawString(ofToString(s, 0), s/60.0 * ofGetWidth()/2 + 4, ofGetHeight()/2 - 4);
verdana.drawString(ofToString(m, 0), m/60.0 * ofGetWidth()/2 + 4, ofGetHeight()/3 - 4);
verdana.drawString(ofToString(h, 0), h/24.0 * ofGetWidth()/2 + 4, ofGetHeight()/6 - 4);
}
//--------------------------------------------------------------
void ofApp::keyPressed(int key){
}
//--------------------------------------------------------------
void ofApp::keyReleased(int key){
}
//--------------------------------------------------------------
void ofApp::mouseMoved(int x, int y ){
}
//--------------------------------------------------------------
void ofApp::mouseDragged(int x, int y, int button){
}
//--------------------------------------------------------------
void ofApp::mousePressed(int x, int y, int button){
mouse_pressed = true;
}
//--------------------------------------------------------------
void ofApp::mouseReleased(int x, int y, int button){
}
//--------------------------------------------------------------
void ofApp::mouseEntered(int x, int y){
}
//--------------------------------------------------------------
void ofApp::mouseExited(int x, int y){
}
//--------------------------------------------------------------
void ofApp::windowResized(int w, int h){
}
//--------------------------------------------------------------
void ofApp::gotMessage(ofMessage msg){
}
//--------------------------------------------------------------
void ofApp::dragEvent(ofDragInfo dragInfo){
}
#pragma once
#include "ofMain.h"
class ofApp : public ofBaseApp{
public:
void setup();
void update();
void draw();
void keyPressed(int key);
void keyReleased(int key);
void mouseMoved(int x, int y );
void mouseDragged(int x, int y, int button);
void mousePressed(int x, int y, int button);
void mouseReleased(int x, int y, int button);
void mouseEntered(int x, int y);
void mouseExited(int x, int y);
void windowResized(int w, int h);
void dragEvent(ofDragInfo dragInfo);
void gotMessage(ofMessage msg);
ofTrueTypeFont verdana;
};
アナログ時計について
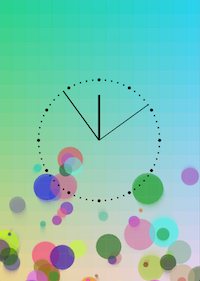
これは、時間を取得したのち、それを針に反映させました。
ソースコードはこちらから
#include "ofApp.h"
int w = 1000;
int ht = 700;
void ofApp::setup(){
ofSetBackgroundAuto(false);
ofSetFrameRate(60);
ofSetWindowShape(w, ht);
ofBackground(250, 250, 250);
ofEnableAlphaBlending();
ofEnableSmoothing();
}
void ofApp::update(){
}
void ofApp::draw(){
int s = ofGetSeconds();
int m = ofGetMinutes();
int h = ofGetHours();
float clockSize = 150;
ofSetColor(0, 0, 0);
//座標全体を中心に移動
ofTranslate(750, ht/2);
//分の目盛を描く
for (int i=0; i<60; i++) {
ofRotateZDeg(6);
ofDrawCircle(clockSize, 0, 2);
}
//時の目盛を描く
for (int i=0; i<12; i++) {
ofRotateZDeg(30);
ofDrawCircle(clockSize, 0, 4);
}
ofPushMatrix();
ofRotateZDeg(s*6.0);
ofSetLineWidth(1);
ofDrawLine(0, 0, 0, -clockSize);
ofPopMatrix();
ofPushMatrix();
ofRotateZDeg(m*6.0);
ofSetLineWidth(2);
ofDrawLine(0, 0, 0, -clockSize);
ofPopMatrix();
ofPushMatrix();
ofRotateZDeg(h*30.0);
ofSetLineWidth(4);
ofDrawLine(0, 0, 0, -clockSize*0.75);
ofPopMatrix();
}
//--------------------------------------------------------------
void ofApp::keyPressed(int key){
}
//--------------------------------------------------------------
void ofApp::keyReleased(int key){
}
//--------------------------------------------------------------
void ofApp::mouseMoved(int x, int y ){
}
//--------------------------------------------------------------
void ofApp::mouseDragged(int x, int y, int button){
}
//--------------------------------------------------------------
void ofApp::mousePressed(int x, int y, int button){
mouse_pressed = true;
}
//--------------------------------------------------------------
void ofApp::mouseReleased(int x, int y, int button){
}
//--------------------------------------------------------------
void ofApp::mouseEntered(int x, int y){
}
//--------------------------------------------------------------
void ofApp::mouseExited(int x, int y){
}
//--------------------------------------------------------------
void ofApp::windowResized(int w, int h){
}
//--------------------------------------------------------------
void ofApp::gotMessage(ofMessage msg){
}
#pragma once
#include "ofMain.h"
class ofApp : public ofBaseApp{
public:
void setup();
void update();
void draw();
void keyPressed(int key);
void keyReleased(int key);
void mouseMoved(int x, int y );
void mouseDragged(int x, int y, int button);
void mousePressed(int x, int y, int button);
void mouseReleased(int x, int y, int button);
void mouseEntered(int x, int y);
void mouseExited(int x, int y);
void windowResized(int w, int h);
void dragEvent(ofDragInfo dragInfo);
void gotMessage(ofMessage msg);
};
丸のインタラクションについて
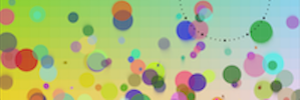
これはマウスによるアクションイベントをシステムに組み込んでいます。
クリックすると、そのカーソルの位置に全ての丸が集まるようにしています。
ソースコードはこちらから
#include "ofApp.h"
static const int NUM = 100;
float loc_x[NUM];
float loc_y[NUM];
float speed_x[NUM];
float speed_y[NUM];
float radius[NUM];
int red[NUM];
int green[NUM];
int blue[NUM];
bool mouse_pressed;
bool mouse_moved;
float gravity;
float friction;
int w = 1000;
int ht = 700;
//--------------------------------------------------------------
void ofApp::setup(){
ofSetBackgroundAuto(false);
ofSetFrameRate(60);
ofSetWindowShape(w, ht);
ofBackground(250, 250, 250);
ofEnableAlphaBlending();
ofEnableSmoothing();
mouse_pressed = false;
mouse_moved = false;
gravity = 0.1;
friction = 0.999;
for(int i = 0; i < NUM; i++){
loc_x[i] = ofRandom(0, ofGetWidth());
loc_y[i] = ofRandom(0, ofGetHeight());
speed_x[i] = ofRandom(-5, 5);
speed_y[i] = ofRandom(-5, 5);
radius[i] = ofRandom(4, 40);
red[i] = ofRandom(0, 255);
green[i] = ofRandom(0, 255);
blue[i] = ofRandom(0, 255);
}
}
//--------------------------------------------------------------
void ofApp::update(){
for(int i = 0; i < NUM; i++){
if(mouse_pressed){
speed_x[i] = (mouseX - loc_x[i]) / 8.0;
speed_y[i] = (mouseY - loc_y[i]) / 8.0;
}
speed_x[i] = speed_x[i] * friction;
speed_y[i] = speed_y[i] * friction;
speed_y[i] = speed_y[i] + gravity;
loc_x[i] = loc_x[i] + speed_x[i];
loc_y[i] = loc_y[i] + speed_y[i];
if(loc_x[i] < 0){
speed_x[i] = speed_x[i] * -1;
}
if(loc_x[i] > ofGetWidth()){
speed_x[i] = speed_x[i] * -1;
}
if(loc_y[i] < 0){
speed_y[i] = speed_y[i] * -1;
}
if(loc_y[i] > ofGetHeight()){
speed_y[i] = speed_y[i] * -1;
}
}
}
//--------------------------------------------------------------
void ofApp::draw(){
int s = ofGetSeconds();
int m = ofGetMinutes();
int h = ofGetHours();
float x;
float y;
float w1;
float h1;
int i,j;
w1 = ofGetWidth()/30.0 + 1;
h1 = ofGetHeight()/20.0 + 1;
x = 0;
y = 0;
for(j = 0; j < 20; j++){
for(i = 0; i < 30; i++){
ofSetColor(255/ 20 * j, 200, 255/30 * i, 127);
x = ofGetWidth() / 30.0 * i;
y = ofGetHeight() /20.0 * j;
ofDrawRectangle(x, y, w1, h1);
}
}
ofSetColor(255, 255, 255, 23);
ofDrawRectangle(0, 0, ofGetWidth(), ofGetHeight());
for(i = 0; i < NUM; i++){
ofSetColor(red[i], green[i], blue[i], 127);
ofDrawCircle(loc_x[i], loc_y[i], radius[i]);
}
}
//--------------------------------------------------------------
void ofApp::keyPressed(int key){
}
//--------------------------------------------------------------
void ofApp::keyReleased(int key){
}
//--------------------------------------------------------------
void ofApp::mouseMoved(int x, int y ){
mouse_moved = true;
gravity = 0.1;
friction = 0.999;
for(int i = 0; i < NUM; i++){
loc_x[i] = ofRandom(0, ofGetWidth());
loc_y[i] = ofRandom(0, ofGetHeight());
speed_x[i] = ofRandom(-1, 5);
speed_y[i] = ofRandom(-1, 5);
radius[i] = ofRandom(4, 40);
red[i] = ofRandom(100, 255);
green[i] = ofRandom(100, 255);
blue[i] = ofRandom(100, 255);
}
}
//--------------------------------------------------------------
void ofApp::mouseDragged(int x, int y, int button){
}
//--------------------------------------------------------------
void ofApp::mousePressed(int x, int y, int button){
mouse_pressed = true;
}
//--------------------------------------------------------------
void ofApp::mouseReleased(int x, int y, int button){
mouse_pressed = false;
for(int i = 0; i < NUM; i++){
speed_x[i] = ofRandom(-5, 5);
speed_y[i] = ofRandom(-5, 5);
red[i] = ofRandom(0, 255);
green[i] = ofRandom(0, 255);
blue[i] = ofRandom(0, 255);
}
}
//--------------------------------------------------------------
void ofApp::mouseEntered(int x, int y){
}
//--------------------------------------------------------------
void ofApp::mouseExited(int x, int y){
}
//--------------------------------------------------------------
void ofApp::windowResized(int w, int h){
}
//--------------------------------------------------------------
void ofApp::gotMessage(ofMessage msg){
}
//--------------------------------------------------------------
void ofApp::dragEvent(ofDragInfo dragInfo){
}
#pragma once
#include "ofMain.h"
class ofApp : public ofBaseApp{
public:
void setup();
void update();
void draw();
void keyPressed(int key);
void keyReleased(int key);
void mouseMoved(int x, int y );
void mouseDragged(int x, int y, int button);
void mousePressed(int x, int y, int button);
void mouseReleased(int x, int y, int button);
void mouseEntered(int x, int y);
void mouseExited(int x, int y);
void windowResized(int w, int h);
void dragEvent(ofDragInfo dragInfo);
void gotMessage(ofMessage msg);
};
今回は時計を作るにあたって、こちらのサイトを参考にさせていただきました。
ありがとうございました。
openFrameworks for iPhone:時計をつくる
時計と連動したアニメーションをOpenframeworksで制作する